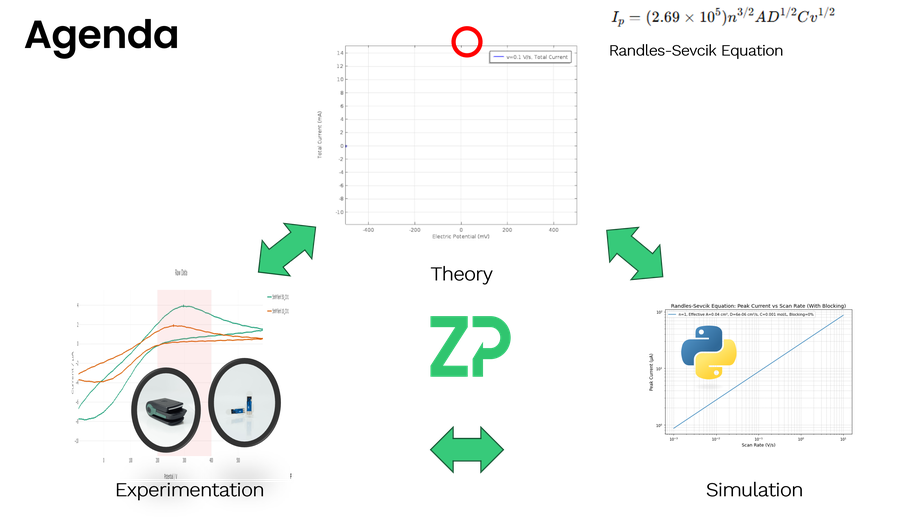
# -*- coding: utf-8 -*-
"""
Created on Sun Jan 5 10:50:40 2025
@author: martp
"""
import numpy as np
import matplotlib.pyplot as plt
# Constants
n = 1 # Number of electrons transferred
A = 0.0416 # Electrode area in cm²
D = 6.0e-6 # Diffusion coefficient in cm²/s
C_mol_L = 5e-3 # Concentration in mol/L
blocking = 0.0 # Fraction of the electrode area blocked (0.5 means 50% blocked)
F = 96485 # Faraday constant in C/mol
R = 8.314 # Gas constant in J/(mol*K)
T = 298 # Temperature in K
# Convert concentration from mol/L to mol/cm³
C = C_mol_L / 1000 # 1 L = 1000 cm³
# Adjust the effective electrode area due to blocking
effective_area = A * (1 - blocking) # Effective area after blocking
# Randles-Sevcik constant at 298 K
k = 2.69e5
# Scan rates in V/s (logarithmic range for better visualization)
v = np.logspace(-3, 1, 500) # From 0.001 to 10 V/s
# Calculate peak current using Randles-Sevcik equation and convert to microamps
Ip_microA = k * n**1.5 * effective_area * D**0.5 * C * v**0.5 * 1e6 # Convert A to µA
# Plot
plt.figure(figsize=(8, 6))
plt.plot(v, Ip_microA, label=f'n={n}, Effective A={effective_area:.2f} cm², D={D} cm²/s, C={C_mol_L} mol/L, Blocking={blocking*100:.0f}%')
plt.xscale('log') # Logarithmic scale for scan rate
plt.yscale('log') # Logarithmic scale for peak current
plt.xlabel('Scan Rate (V/s)', fontsize=12)
plt.ylabel('Peak Current (µA)', fontsize=12)
plt.title('Randles-Sevcik Equation: Peak Current vs Scan Rate (With Blocking)', fontsize=14)
plt.grid(which='both', linestyle='--', linewidth=0.5)
plt.legend(fontsize=10)
plt.tight_layout()
plt.show()